How to prepare Django to tango with Vue
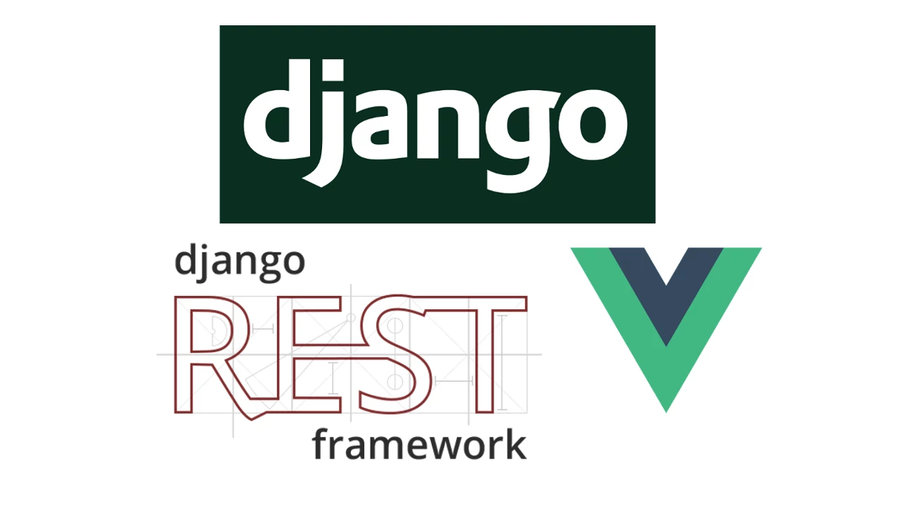
This is how I have setup and prepared a Django app to tango with Vue.js as front-end.
The next post will be a post about how to get Vue to tango with Django.
This post is focusing on the Django backend.
I used Django, Django REST Framework, Vue, VueResource, VueRouter, NPM and Webpack.
You can find the more complete and extensive source code at my Github.
I assume that you know how to setup and work whit django,
otherwise I recommend that you begin looking at the official Django tutorial.
Back-end
Create your app
python manage.py startapp gallery
Django Model and Admin
The model.py and admin.py code we need in our app look like this.
model.py
from django.db import models
class Art(models.Model):
art_file = models.ImageField(upload_to='gallery')
pub_date = models.DateTimeField(blank=True, null=True))
title = models.CharField(max_length=50, blank=True, null=True)
class Meta:
ordering = ['pk']
def __unicode__(self):
if self.title:
return u'%s' % self.title
return u'%s' % self.pk
def __str__(self):
if self.title:
return u'%s' % self.title
return u'%s' % self.pk
admin.py
from django.contrib import admin
from .models import Art
admin.site.register(Art)
Django REST-Framework
Now if you have a Django app setup and running you need a backend API
to get data from to the front-end.
Django REST framework is perfect for this.
We will follow the steps in the Django REST framework tutorial but I will use our models and code as examples,
so that we have that setup for the front-end part of this how to.
Begin to install it with:
pip install djangorestframework
add this to your settings.py file.
INSTALLED_APPS = (
'rest_framework',
'gallery',
)
REST_FRAMEWORK = {
'DEFAULT_PERMISSION_CLASSES': [
'rest_framework.permissions.DjangoModelPermissionsOrAnonReadOnly'
],
'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.PageNumberPagination',
'PAGE_SIZE': 10,
'DEFAULT_RENDERER_CLASSES': (
'rest_framework.renderers.JSONRenderer',
'rest_framework.renderers.BrowsableAPIRenderer'
)
}
and run the management command:
python manage.py migrate
Serialize models
We need to serialize our models to something the fron-end can handle.
Create a serializer.py file in the django app folder and add this code:
from rest_framework import serializers
from .models import Art
class ArtSerializer(serializers.ModelSerializer):
class Meta:
model = Art
fields = ('pk', 'art_file', 'title')
API views
Now we need an API/view which return the serialized models to the front-end.
Add to your view.py file this view code:
from rest_framework.viewsets import ReadOnlyModelViewSet
from .models import Art
from .serializers import ArtSerializer
class ArtViewSet(ReadOnlyModelViewSet):
queryset = Art.objects.all()
serializer_class = ArtSerializer
API urls
In order to access the views there is a need to setup the urls.
First setup routers.
Create a routers.py file in the django app folder and add this code:
from rest_framework import routers
from .views import ArtViewSet
gallery_router = routers.DefaultRouter()
gallery_router.register(r'art', ArtViewSet)
To setup the urls add this code to the urls.py file.
from django.conf.urls import url, include
from .routers import gallery657_router
urlpatterns = [
url(r'^api/', include(gallery_router.urls)),
]
Now you should be able to browse the API at /gallery/api/.
From this API it's now also possible for any front-end to get the data.
References:
[1]: django-rest-framework tutorial Retrieved: 22 April 2017 12:00 django-rest-framework quickstart tutorial
[2]: Vue-router 2 - Getting Started Retrieved: 27 Augusti 2017 18:00 Vue-router 2 - Getting Started tutorial